I have a game with several background tilesprites, some tileset layers, as well …as a few sprites and text objects on screen. I am seeing a blue artifact effect appear at various locations on the screen. I'm using Phaser v3.54.0, and I've tested also reproduced the effect with other versions (I went backward until 3.2x, where the code no longer ran).
Unfortunately my phone security settings forbid screenshots, but here's what it looks like:
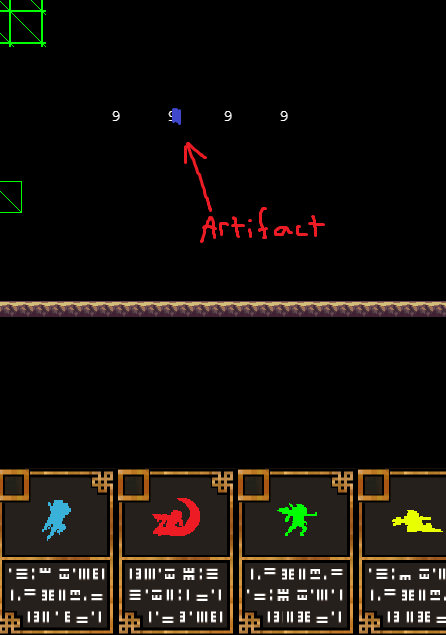
Here's a summary of the effect:
• Occurs on Android devices (haven't tried iPhone), not on desktop (even in mobile mode)
• Appears in all Android browsers (tried 3 different Android phones)
• Only occurs in WEBGL, not in CANVAS
• Tends to occur along edges of sprites, text, and tiles (shooting a fireball causes the effect to appear all around the player)
• Appears at predictable times and locations (i.e. the same places and times every playthrough, other things constant)
• Changing camera and game object properties (depth, alpha, position) causes the effect to appear at different times and in different locations
After removing everything I could while retaining the effect. I was left with the code below. Here's a summary of what I've found in this example:
• Removing the tilesprites, tilemap layer, images, or text objects causes the effect to disappear
• Failing to preload the background images or player sprite has no impact, but failing to preload the card images, or changing their image source, changes or removes the effect
• Changing the background tilesprite size or location has no effect
• Changing the tilemap size or content has no effect
Here is the example code:
var _game = {
mapIndex: null,
platforms: null,
player: null
};
_game.playScene = new Phaser.Scene('play');
var config = {
type: Phaser.AUTO,
parent: 'game',
width: 450,
height: 800,
pixelArt: true, // Removing pixelArt changes the artifact pattern
scale: {
},
scene: [
_game.playScene
],
physics: {
default: 'arcade',
arcade: {
debug: _game.debugging
}
}
};
_game.game = new Phaser.Game(config);
_game.game.scene.start('play', { map: 0 });
_game.playScene.init = function(data) {
_game.mapIndex = data.map;
};
_game.playScene.preload = function() {
this.load.image('cloudback', 'img/CloudsBack.png');
this.load.image('cloudfront', 'img/CloudsFront.png');
this.load.image('bgback', 'img/BGBack.png');
this.load.image('bgfront', 'img/BGFront.png');
this.load.image('tileset', 'img/Tileset.png');
this.load.tilemapCSV('map_platforms' + _game.mapIndex, 'lib/' + _game.mapIndex + '_Platforms.csv');
this.load.image('cardJump', 'img/CardJump.png');
this.load.image('cardAttack', 'img/CardAttack.png');
this.load.image('cardMagic', 'img/CardMagic.png');
this.load.image('cardDash', 'img/CardDash.png');
this.load.spritesheet('adventurer', 'img/Adventurer.png', { frameWidth: 50, frameHeight: 37});
};
_game.playScene.create = function() {
this.cloudback = this.add.tileSprite(0, 0, 100, 100, 'cloudback');
this.cloudfront = this.add.tileSprite(0, 0, 100, 100, 'cloudfront');
this.bgback = this.add.tileSprite(0, 0, 100, 100, 'bgback');
this.bgfront = this.add.tileSprite(0, 0, 100, 100, 'bgfront');
var map_platforms = this.make.tilemap({ key: 'map_platforms' + _game.mapIndex, tileWidth: 16, tileHeight: 16 });
var pl_tileset = map_platforms.addTilesetImage('tileset');
var pl_layer = map_platforms.createLayer(0, pl_tileset, 0, 0);
_game.player = this.physics.add.sprite(10, 200, 'adventurer');
this.cardJump = this.add.sprite(60, 555, 'cardJump')
this.cardAttack = this.add.sprite(180, 555, 'cardAttack')
this.cardMagic = this.add.sprite(300, 555, 'cardMagic')
this.cardDash = this.add.sprite(420, 555, 'cardDash')
var cardJump = this.add.text(115, 112, 9);
var cardAttack = this.add.text(171, 112, 9);
var cardMagic = this.add.text(227, 112, 9);
var cardDash = this.add.text(283, 112, 9);
};
Thanks for your time!
Duncan Priebe